Add a back button to your Next.js app
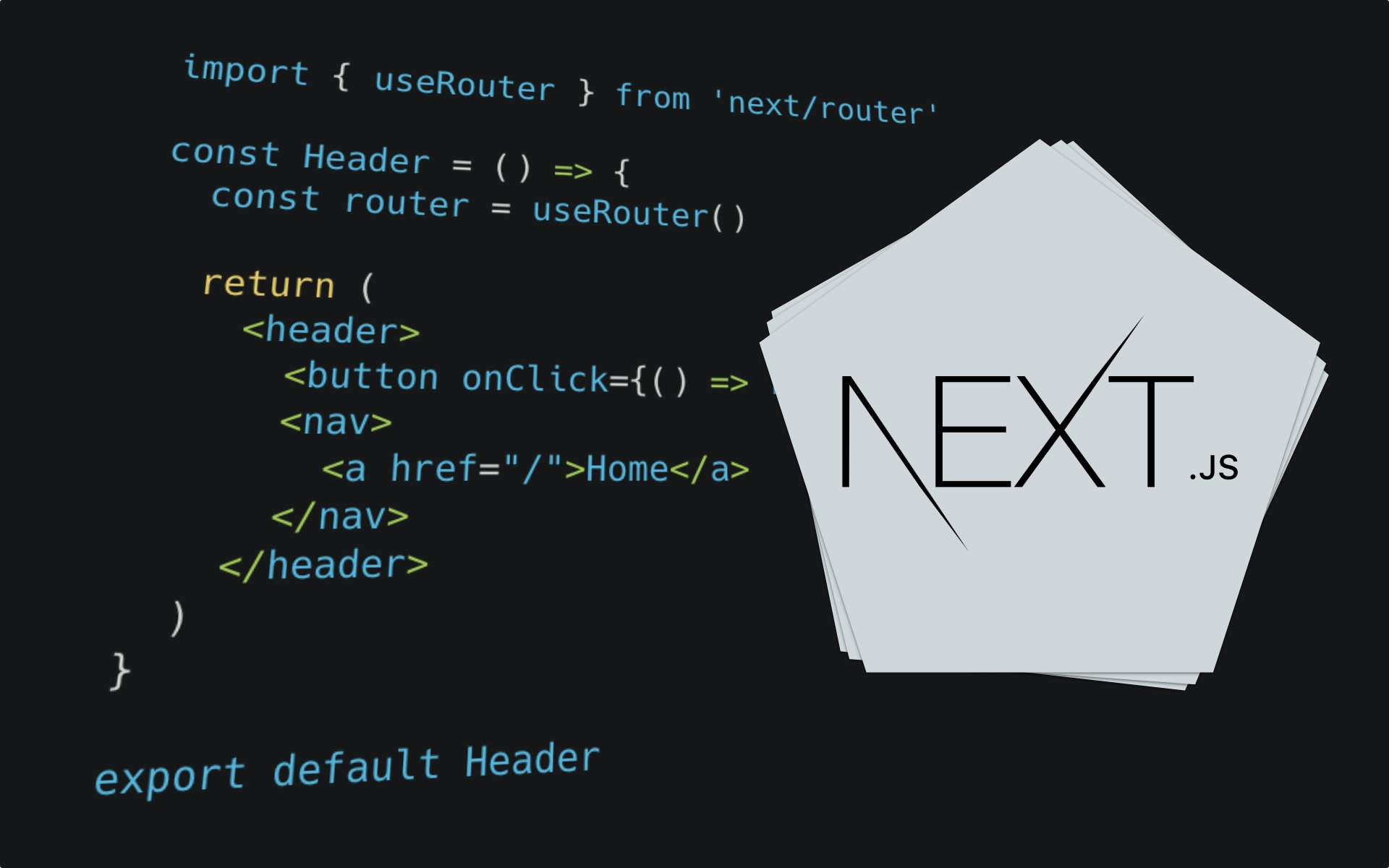
This tutorial will walk you through the steps to add a simple back button to your Next.js project, offering a more app-like experience by allowing users to navigate to the previous page with just a click.
Setup
If you have an existing project then you can skip the setup steps but if you're starting from scratch you can create a new project in two ways, either buy manually creating the files or using npx. A full setup guide can be found in the Next.js docs if you'd prefer to read it in full.
Use npx to scaffold a project
This will create a basic project using the Next.js cli with npx - meaning you won't need to install next-cli globally on your computer.
npx create-next-app
# or
yarn create next-app
Manual setup
You can also create a project from scratch, which is what I personally prefer to do, and add everything you need manually. This can be done in three simple steps.
- Run
npm init
to create a new package.json file, you can name the project whatever you like and go through each step adding whatever info you need. Once created, open package.json and add the following scripts:
"scripts": {
"dev": "next dev",
"build": "next build",
"start": "next start"
}
A short explanation of what each script does:
dev - runs next dev which starts Next.js in development mode
build - runs next build which builds the application for production usage
start - runs next start which starts a Next.js production server
- Install next, react and react-dom in your project:
npm install next react react-dom
# or
yarn add next react react-dom
- Create a folder named 'pages' in the root of your application. Inside the folder create a file named 'index.js' and add the code below. By default, any file using the .js extension added to the the pages folder in the root of an application will be served as a page. Visiting '/' or '/index' in your application will display the page.
function HomePage() {
return (
<div>
<h1>Homepage</h1>
</div>
)
}
export default HomePage
Next.js Router
Adding a back button to you project is really easy with Next.js, by default, Next comes with access to a router. You can import it and use it from the next package using the following syntax:
import { useRouter } from "next/router"
const router = useRouter()
This gives you access to the router object, including information such as:
- pathname: [String] - Current route. That is the path of the page in '/pages'
- query: [Object] - The query string parsed to an object. It will be an empty object during prerendering if the page doesn't have data fetching requirements.
- asPath: [String] - The path (including the query) shown in the browser without the configured basePath or locale.
- basePath: [String] - The active basePath (if enabled).
- isReady: [boolean] - Whether the router fields are updated client-side and ready for use.
You can also access router methods such as router.replace()
, router.reload()
or router.back()
, which is what we'll need for the back button.
Add button to your project
You can add the back button anywhere in your project, but for this tutorial we'll create a header component and add the button there, this will allow you to re-use the button on each page of your app by importing the header. You can create a 'components' folder in the root of your project and add a Header.js file there.
import { useRouter } from "next/router"
const Header = () => {
const router = useRouter()
return (
<header>
<button onClick={() => router.back()}>Back</button>
<nav>
<a href="/">Home</a>
</nav>
</header>
)
}
export default Header
This is just a simple example of a header with navigation tags and a link to the homepage, you can add to this and style it however you like but for this tutorial a simple header component will be enough.
You could also go further and wrap the button in a conditional statement that only displays the back button on pages other than the homepage.
<header>
{router.pathname !== "/" && (
<button onClick={() => router.back()}>Back</button>
)}
<nav>
<a href="/">Home</a>
</nav>
</header>
The above code compares the current URL path (extracted from the router object) with the homepage path '/' and only shows the button if they are not equal.
Open the home page component and import the newly created 'Header.js' componenet with your back button.
import Header from "../components/Header"
function HomePage() {
return (
<div>
<Header />
<h1>Homepage</h1>
</div>
)
}
export default HomePage
You now have access to the back button on every page of your app. This is a simple example of how easy it is to add functionality such as a back button to your project using the Next.js API features.